Do you know that date handling is an integral aspect of web development, particularly while building applications that necessitate user input for dates? So, effective date handling helps make sure that your application is reliable, accurate, and user-friendly. If you are looking forward to hiring a professional React JS development company, then first of all, you should get a good idea about date handling in ReactJS along with ReactJS DatePicker. We will discuss all these details comprehensively in this guide. So, let’s dig in:
What is Referred to as Data Handling in ReactJS?
Before diving deep into DataPickers, it becomes highly important for you to get a good idea of how date handling functions in ReactJS. In this regard, it is crucial to note that JavaScript offers native data objects. However, meticulous consideration is required to manage those within React components.
There are many challenges that one should focus on addressing to ensure a smooth user experience. Some of these entail formatting, time zones, and user input validation. Make sure to hire ReactJS developers to get expert assistance.
What are ReactJS DatePickers?
DatePickers typically refers to UI components that are designed for the purpose of streamlining data selection for users. ReactJS is known for providing multiple components and libraries for the implementation of DataPickers. Again, each of these possesses its own set of customization alternatives and features. Now, it is time to have a quick look at some of the well-known ReactJS DatePicker libraries. We will also demonstrate how to integrate those into your projects. So, let’s find out:
React Datepicker
React Datepicker is a versatile and lightweight DatePicker library for React applications. It is known for providing comprehensive support for different data formats, keyword navigation, and localization. Let’s have a look at a basic example of employing DatePicker ReactJS in a React component:
import React, { useState } from ‘react’;
import ‘react-datepicker/dist/react-datepicker.css’;
const MyDatePicker = () => {
const [selectedDate, setSelectedDate] = useState(null);
return (
setSelectedDate(date)}
/>
);
};
export default MyDatePicker;
“`
▲
In this case, you will see that the `DatePicker` component is imported from the `react-datepicker` library. Also, rendering of it occurs within a functional component. Here, we have used React’s `useState` hook for proper management of the chosen date state.
React Datepicker is involved in providing a diverse selection of customization alternatives which includes localization, data formatting, and date range selection. You can effortlessly configure it to ensure that it caters to the specific requirements of your app. Configuration can be done easily when you hire professional ReactJS developers inclined towards offering cutting-edge React JS development solutions.
Material-UI DatePicker
Material-UI is a recognized React UI framework that offers a detailed set of components that entails a DatePicker. The Material-UI DatePicker provides a contemporary design, broad customization alternatives, and smooth integration with other Material-UI components. Now, let’s have a look at the best ways in which you can leverage Material-UI’s DatePicker ReactJS component, especially in a React component:
import React, { useState } from ‘react’;
const MyDatePicker = () => {
const [selectedDate, handleDateChange] = useState(new Date());
return ();
};
export default MyDatePicker;
“`
▲
In this ReactJS DatePicker example, the `DatePicker` component is imported generally from Material-UI’s `@material-ui/pickers` package. After that, rendering of it occurs within a functional component. Also, in this case, React’s `useState` hook is used for effectively managing the chosen date state and the `handleDateChange` function for updating the selected date.
Material-UI DatePicker is known for providing a vast array of customization alternatives which includes localization, data formats, and data range selection. You can smoothly integrate it into your Material-UI-based applications and tailor it so that it effectively matches the design aesthetic of your application. To learn more, you can reach out to a professional React JS development company.
Ant Design DatePicker
Ant Design is another well-known UI library that is designed for React. It provides a DatePicker component with a sleek design and comes with a lot of customization options. For more details, you can hire ReactJS developers. Now, it is the time to have a look at how to make use of the Ant Design’s DatePicker component, particularly in a React component:
import React, { useState } from ‘react’;
import ‘antd/dist/antd.css’;
const MyDatePicker = () => {
const [selectedDate, setSelectedDate] = useState(null);
return (
setSelectedDate(date)}
/>
);
};
export default MyDatePicker;
“`
▲
Here, you will observe that we have imported the `DatePicker` component from the `antd` library. We also have rendered it within a functional component. Also, we have leveraged React’s `useState` hook for effective management of the chosen date state.
With support for different date formats, date range selection, and localization, Ant Design DatePicker ReactJS is known for providing an intuitive and contemporary date selection experience. It smoothly integrates with other Ant Design components and can be easily tailored to match the design requirements of your application.
React-Datetime
React-Datetime is known for its incredible flexibility. It is a customizable DatePicker library for React applications. Whenever it comes to date selection, it offers an intuitive and simple interface. It also provides support for different date formats, data range picking, and time selection. Let’s find out how to leverage React-Datetime in a React component before you choose ReactJS developers:
import React, { useState } from ‘react’;
import ‘react-datetime/css/react-datetime.css’;
const MyDatePicker = () => {
const [selectedDate, setSelectedDate] = useState(null);
return (
setSelectedDate(date)}
/>
);
};
export default MyDatePicker;
“`
▲
This example also shows the import of the Datetime component from the `react-datetime` library. It is further rendered within a functional component. In this case, React’s `useState` hook is employed for the appropriate management of the chosen date state.
React-Datetime again provides several customization alternatives which entail time and date formatting, localization along disabling specific dates. It again offers a smooth date selection experience, particularly for users. Not only that, but one can also effortlessly integrate it into your React applications. If you need any assistance with anything related, you can contact a reputed React JS development company.
React-DateRangePicker
Another popular and versatile DatePicker library for React applications includes React-DateRangePicker. It lets users choose date ranges. Along with that, it provides an intuitive and modern interface for choosing start and end dates with support for different customization alternatives. Now, let’s find out how to effectively leverage React-DateRangePicker in a React component before you hire ReactJS developers:
import React, { useState } from ‘react’;
import ‘react-date-range/dist/styles.css’; // main css file
import ‘react-date-range/dist/theme/default.css’; // theme css file
const MyDatePicker = () => {
const [selectedRange, setSelectedRange] = useState([
{
startDate: new Date(),
endDate: null,
key: ‘selection’
}
]);
return (
setSelectedRange([ranges.selection])}
/>
);
};
export default MyDatePicker;
“`
▲
Similar to the above examples, in this case, there is the import of the `DateRangePicker` component from the `react-date-range` library. After that, the rendering of it is done within a functional component. Finally, React’s `useState` hook is employed to effectively manage the chosen date range state.
React-DateRangePicker is known for providing multiple customization alternatives, which include date formatting, custom date range presets, and styling. Also, it offers a streamlined date range selection experience for users. You can even effortlessly integrate it into your React applications.
React-DatePicker
React-DatePicker is a highly configurable DatePicker library for React applications. It offers a simple and intuitive interface for date selection, with support for various customization options. Let’s see how to use React-DatePicker in a React component:
import React, { useState } from ‘react’;
import ‘react-datepicker/dist/react-datepicker.css’;
const MyDatePicker = () => {
const [selectedDate, setSelectedDate] = useState(null);
return (
setSelectedDate(date)}
/>
);
};
export default MyDatePicker;
“`
▲
Similar to the above examples, in this case, there is the import of the `DateRangePicker` component from the `react-date-range` library. After that, the rendering of it is done within a functional component. Finally, React’s `useState` hook is employed to effectively manage the chosen date range state.
Similar to the other discussed options, React-DatePicker provides multiple customization options from date range selection to date formatting. It even offers a smooth date selection experience for users which can be effortlessly integrated into your React applications.
Carbon DatePicker
The final name in our list is Carbon DatePicker, which refers to a React component library developed by IBM that offers a sleek and contemporary DatePicker component. It offers support for different data formats, localization, along with accessibility features. Now, let’s find out how to make use of a Carbon DatePicker in a React component:
import React, { useState } from ‘react’;
import ‘carbon-react/lib/__experimental__/components/date-picker/date-picker.scss’;
const MyDatePicker = () => {
const [selectedDate, setSelectedDate] = useState(null);
return (
setSelectedDate(date)}
/>
);
};
export default MyDatePicker;
“`
▲
In this case, the import of the `DatePicker` component is done from the `carbon-react` library. After that, it is rendered especially within a functional component. Finally, React’s `useState` hook is employed for managing the chosen date state.
With support for different accessibility features like keyboard navigation and screen reader compatibility, Carbon DatePicker provides an intuitive and modern date selection experience. It smoothly integrates with other Carbon components and can be effortlessly tailored to suit the design requirements of your application. To learn how to use DatePicker in ReactJS, all that you need is to reach out to a professional ReactJS development agency,
Final Verdict
Now that we have explored some of the most popular DatePicker libraries for ReactJS, it is important to note that each of the libraries provides unique features and customization alternatives. Thus, it allows you to come up with an intuitive and robust date selection experience for your users. So, if you are looking forward to building reliable and user-friendly applications, mastering date handling in ReactJS is considered to be an absolutely important step.
Irrespective of whether you are crafting a simple form or a complicated scheduling application, it becomes integral to get a good know-how of the most effective date-handling techniques. Also, you should implement these well in order to deliver user-friendly and high-quality applications in ReactJS. So, don’t hesitate to experiment with various DatePicker libraries and customization options by choosing to hire dedicated ReactJS developers.
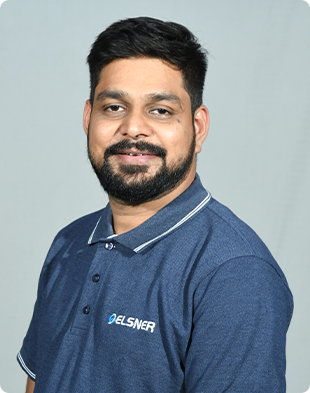
About Author
Tarun Bansal - Technical Head
Tarun is a technology enthusiast with a flair for solving complex challenges. His technical expertise and deep knowledge of emerging trends have made him a go-to person for strategic tech initiatives. Passionate about innovation, Tarun continuously explores new ways to drive efficiency and performance in every project he undertakes.